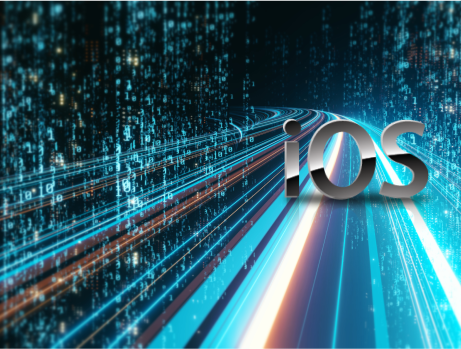
Quick actions was made by Apple for iOS 12 and higher versions. These actions allow the user to make actions without getting into the app itself. It saves time on common actions and makes user interface easier and quicker. These actions work by long pressing the app in the menu screen or by 3D touching the app (if device support 3D Touch).
There are two types of shortcut items:
- Static type – defined in the info.plist.
- Dynamic type – defined while the app is running or, more common, when the app resign active.
A source code example:
https://github.com/msmobileapps/Quick-Actions-IOS-Blog
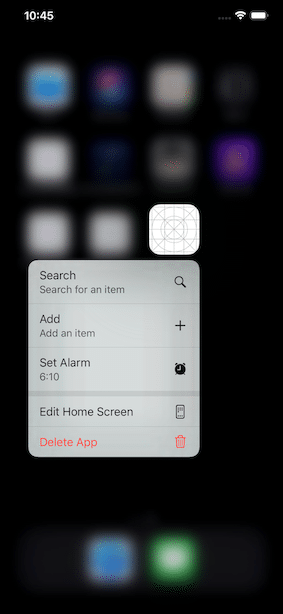
The Static type
First, in order to set up these actions in your app, you will have to go to the info.plist and add the following properties:
- Add UIApplicationShortcutItems array with the number of shortcut item you want.
- Set each item to Dictionary type.
- Add the properties:
- UIApplicationShortcutItemType – A unique string that identifies the action.
- UIApplicationShortcutItemTitle – The title that displayed to the user.
- UIApplicationShortcutItemSubtitle – The subtitle that displayed to the user.
- UIApplicationShortcutItemIconType – Set an Apple predicate icon (optional).
- UIApplicationShortcutItemIconFile – Set a custom icon (optional).
- UIApplicationShortcutItemUserInfo – dictionary with saved data.
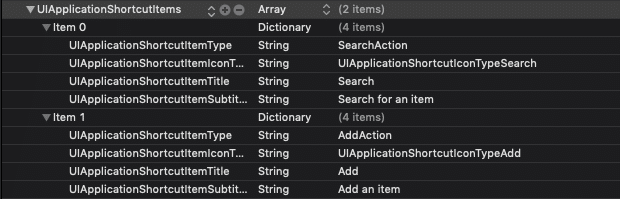
Second, go to the AppDelegate. Add a parameter of type UIApplicationShortcutItem. Then in didFinishLaunchingWithOptions functions add the following code:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
if let shortcutItem = launchOptions?[UIApplication.LaunchOptionsKey.shortcutItem] as? UIApplicationShortcutItem {
shortcutItemToProcess = shortcutItem
}
return true
}
These lines of code check if there is a value for shortcut item key in launchOptions dictionary. In other words, it checks if the app launched by pressing on a quick shortcut. If true, save this shortcut to our UIApplicationShortcutItem parameter.
In the performActionFor shortcutItem function add the code below. This function is called if the app was still in memory and the shortcut item was triggered.
func application(_ application: UIApplication, performActionFor shortcutItem: UIApplicationShortcutItem, completionHandler: @escaping (Bool) -> Void) {
shortcutItemToProcess = shortcutItem
}
Last, in the applicationDidBecomeActive function add a handling for the shortcuts after they are being pressed.
func applicationDidBecomeActive(_ application: UIApplication) {
if let shortcutItem = shortcutItemToProcess {
var message = ""
switch shortcutItem.type {
case "com.msapps.QuickActions.SearchAction":
message = "Search action was chosen"
case "com.msapps.QuickActions.AddAction":
message = "Add action was chosen"
default:
break
}
let alertController = UIAlertController(title: "Quick Action Selected", message: message, preferredStyle: .alert)
alertController.addAction(UIAlertAction(title: "Close", style: .default, handler: nil))
DispatchQueue.main.async { [unowned self] in
self.window?.rootViewController?.present(alertController, animated: true, completion: nil)
}
//reset the shortcut item
shortcutItemToProcess = nil
}
}
In this example the app shows an alert for each action, but you can choose to do any actions you would like. Also, as you noticed, in the end of the function we set the UIApplicationShortcutItem parameter back to nil to reset it and prevent any possible problems.
The Dynamic type
In order to do a dynamic shortcut, add in applicationWillResignActive function add a shortcut items array to the shortcutItems parameter of the application. You can initialise the item with a type and a string title or with a subtitle, an icon and user info dictionary as well.
An example code:
func applicationWillResignActive(_ application: UIApplication) {
application.shortcutItems =
[UIApplicationShortcutItem(type: "com.msapps.QuickActions.AlarmAction",
localizedTitle: "Set Alarm",
localizedSubtitle: "10:00",
icon: UIApplicationShortcutIcon(type: .alarm),
userInfo: ["Name":"Set Alarm" as NSSecureCoding])]
}
In order to handle actions after the dynamic shortcut was selected, add it’s type to the applicationDidBecomeActive switch.
Read our article about similar issue for android apps – Android Instant App